Processing Development Environment (PDE)
Sketchbook
Tabs, Multiple Files, and Classes
Coordinates
Programming Modes
Rendering Modes
Applet Export
Application Export
Applet Security Restrictions
"Present" Features
Processing Development Environment (PDE)
The Processing Development Environment (PDE) consists of a simple text
editor for writing code, a message area, a text console, tabs for managing
files, a toolbar with buttons for common actions, and a series of menus.
When programs are run, they open in a new window called the display window.
Software written using Processing are called sketches. These sketches are written in the text editor. It has features for cutting/pasting and for searching/replacing text. The message area gives feedback while saving and exporting and also displays errors. The console displays text output by Processing programs including complete error messages and text output from programs with the print() and println() functions. The toolbar buttons allow you to run and stop programs, create a new sketch, open, save, and export:
![]() |
Run Compiles the code, opens a display window, and runs the program inside. Hold down shift to Present instead of Run. |
|
![]() |
Stop Terminates a running program. |
|
![]() |
New Creates a new sketch (project) in the current window. To create a new sketch in its own window, use File → New. |
|
![]() |
Open Provides a menu with options to open files from the sketchbook, open an example, or open a sketch from anywhere on your computer. Opening a sketch from the toolbar will replace the sketch in the current window. To open a sketch in a new window, use File → Open. |
|
![]() |
Save Saves the current sketch to its current location. If you want to give the sketch a different name, select “Save As” from the File menu. |
|
![]() |
Export Exports the current sketch as a Java Applet embedded in an HTML file. The folder containing the files is opened. Click on the index.html file to load the software in the computer's default web browser. Hold down shift to export an application instead of an applet. Note that exporting a sketch will delete the previous contents of the “applet” or “application.xxxx” folders. |
Additional commands are found within the five menus: File, Edit, Sketch, Tools, Help. The menus are context sensitive which means only those items relevant to the work currently being carried out are available.
File
- New (Ctrl+N)
Creates a new sketch in a new window, named as the current date is the format "sketch_YYMMDDa". - Open (Ctrl+O)
Open a sketch in a new window. - Sketchbook
Open a sketch from the sketchbook folder. - Examples
Open one of the examples included with Processing. - Close (Ctrl+W)
Close the sketch in the frontmost window. If this is the last sketch that's open, you will be prompted whether you would like to quit. To avoid the prompt, use Quit instead of Close when you want to exit the application. - Save (Ctrl+S)
Saves the open sketch in it's current state. - Save as... (Ctrl+Shift+S)
Saves the currently open sketch, with the option of giving it a different name. Does not replace the previous version of the sketch. - Export (Ctrl+E)
Exports a Java Applet and creates and embeds it into an HTML file. After the files are exported, the directory containing the exported files is opened. There is more information about exporting here. Note that exporting a sketch will delete the previous contents of the “applet” folder. - Export Application (Ctrl+Shift+E)
Exports as a Java application as an executable file. Opens the directory containing the exported files. Note that exporting a sketch will delete the previous contents of the “application.xxxxx” folders. - Page Setup (Ctrl+Shift+P)
(Not working yet) - Print (Ctrl+P)
(Not working yet) - Preferences (Ctrl+,)
Allows you to change some of the ways Processing works. - Quit (Ctrl+Q)
Exits the Processing Environment and closes all Processing windows.
Edit
- Undo (Ctrl+Z)
Reverses the last command or the last entry typed. Cancel the Undo command by choosing Edit » Redo. - Redo (Ctrl+Y)
Reverses the action of the last Undo command. This option is only available, if there has already been an Undo action. - Cut (Ctrl+X)
Removes and copies selected text to the clipboard (an off-screen text buffer) - Copy (Ctrl+C)
Copies selected text to the clipboard. - Copy for Discourse (Shift+Ctrl+C)
Formats code so that it will appear in the Processing Discourse the same way it appears in the Processing environment and copies it to the clipboard so it can be pasted somewhere else. - Paste (Ctrl+V)
Inserts the contents of the clipboard at the location of the cursor, and replaces any selected text. - Select All (Ctrl+A)
Selects all of the text in the file which is currently open in the text editor. - Comment/Uncomment (Ctrl+/)
Comments the selected text. If the selected text is already commented, it uncomments it. - Increase Indent (Ctrl+])
Indents the selected text two spaces. - Decrease Indent (Ctrl+[)
If the text is indented, removes two spaces from the indent. - Find (Ctrl+F)
Finds an occurance of a text string within the file open in the text editor and gives the option to replace it with a different text. - Find Next (Ctrl+G)
Finds the next occurance of a text string within the file open in the text editor.
Sketch
- Run (Ctrl+R)
Runs the code (compiles the code, opens the display window, and runs the program inside) - Present (Ctrl+Shift+R)
Runs the code in the center of the screen with a neutral background. Click the "stop" button in the lower left to exit the presentation. - Stop
If the code is running, stops the execution. Programs written with the Basic Mode or using the draw() structure are stopped automatically after they draw. - Import Library
Adds the necessary import statements to the top of the current sketch. For example, selecting Sketch » Import Library » video adds the statement "import processing.video.*;" to the top of the file. These import statements are necessary for using the Libraries. - Show Sketch Folder
Opens the directory for the current sketch. - Add File
Opens a file navigator. Select an image, font, or other media files to add it to the sketches "data" directory.
Tools
- Auto Format (Ctrl-T)
Attempts to format the code into a more human-readable layout. Auto Format was previously called Beautify. - Create Font...
Converts fonts into the Processing font format and adds to the current sketch. Opens a dialog box which give options for setting the font, it's size, if it is anti-aliased, and if all characters should be generated. If the "All Characters" options is selected, non-English characters such as ü and Å are generated, but the font file is larger in size. The amount of memory required for the font is also determined by the size selected. Processing fonts are textures, so larger fonts require more image data. - Color Selector
Interface for selecting colors. - Archive Sketch
Archives a copy of the current sketch in .zip format. The archive is placed in the same directory as the sketch. - Fix Encoding and Reload
Sketches that contain non-ASCII characters and were saved with Processing 0140 and earlier may look strange when opened. Garbled text and odd characters may appear where umlauts, cedillas, and Japanese formerly lived. This will reload your sketch using the same method as previous versions of Processing, at which point you can re-save it which will write a proper UTF-8 version.
Help
- Getting Started
Opens the reference for the Processing Environment in the default Web browser. - Troubleshooting
Opens the troubleshooting information in the default Web browser. - Reference
Opens the reference in the default Web browser. Includes reference for the language, programming environment, libraries, and a language comparison. - Find in Reference (Ctrl+Shift+F)
Select a word in your program and select "Find in Reference" to open that reference HTML page. - Frequently Asked Questions
Answers to some basic question about the Processing project. - Visit Processing.org (Ctrl+5)
Opens default Web browser to the Processing.org homepage. - About Processing
Opens a concise information panel about the software.
Sketchbook
All Processing projects are called sketches. Each sketch has its own folder. The main program file for each sketch has the same name as the folder and is found inside. For example, if the sketch is named "Sketch_123", the folder for the sketch will be called "Sketch_123" and the main file will be called "Sketch_123.pde". The PDE file extension is an acronym for the Processing Development Environment.
A sketch folder sometimes contains other folders for media files and code libraries. When a font or image is added to a sketch by selecting "Add File..." from the Sketch menu, a "data" folder is created. Files may also be added to your Processing sketch by dragging them into the text editor. Image and sound files dragged into the application window will automatically be added to the current sketch's "data" folder. All images, fonts, sounds, and other data files loaded in the sketch must be in this folder. Sketches are stored in the Processing folder, which will be in different places on your computer or network depending if you use PC, Mac, or Linux and how the preferences are set. To locate this folder, select the "Preferences" option from the "File" menu (or from the “Processing” menu on the Mac) and look for the "Sketchbook location".
It is possible to have multiple files in a single sketch. These can be Processing text files (the extension .pde) or Java files (the extension .java). To create a new file, click on the arrow button to the right of the file tabs. This button gives access to creating, deleting, and renaming the files that comprise the current sketch. You can write functions and classes in new PDE files and you can write any Java code in files with the JAVA extension. Working with multiple files makes it easier to re-use code and to separate programs into small sub-programs.
Tabs, Multiple Files, and Classes
It can be inconvenient to write a long program within a single file. When programs grow to hundreds or thousands of lines, breaking them into modular units helps manage the different parts. Processing manages files with the Sketchbook and each sketch can have multiple files that are managed with tabs. The arrow button in the upper-right corner of the Processing Development Environment is used to manage these files. Click this button to reveal options to create a new tab, rename the current tab, and delete the current tab. If a project has more than one tab, they can also be hidden and revealed. Hiding a tab temporarily removes that code from the sketch (it will not be compiled with the program when you press Run).
Tabs are intended for more advanced users, and for this reason, the menu that controls the tabs is intentionally made less prominent.
For programmers familiar with Java. When a program with multiple tabs is run, the code is grouped together and the classes in other tabs become inner classes. Because they're inner classes, they cannot have static variables. Simply place the "static" variable outside the class itself to do the same thing (it need not be explicitly named "static" once you list it in this manner). If you don't want code to be an inner class, you can also create a tab with a .java suffix, which means it will be interpreted as straight java code. It is also not possible to use static classes in separate tabs. If you do this, however, you'll need to pass the PApplet object to that object in that tab in order to get PApplet functions like line(), loadStrings() or saveFrame() to work.
Currently, the tabs get truncated when there are too many (Bug 54).
Coordinates
Processing uses a Cartesian coordinate system with the origin in the upper-left corner. If your program is 320 pixels wide and 240 pixels high, coordinate [0, 0] is the upper-left pixel and coordinate [320, 240] is in the lower-right. The last visible pixel in the lower-right corner of the screen is at position [319, 239] because pixels are drawn to the right and below the coordinate.
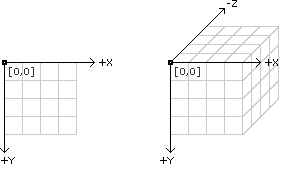
Processing can also simulate drawing in three dimensions. At the surface of the image, the z-coordinate is zero, with negative z-values moving back in space. When drawing in simulated 3D, the "camera" is positioned in the center of the screen.
Programming Modes
Processing allows people to program at three levels of complexity: Basic Mode, Continuous Mode, and Java Mode. People new to programming should begin with the Basic Mode to learn about coordinates, variables, and loops before moving to Continuous and Java modes.
Basic
This mode is used drawing static images and learning fundamentals of programming. Simple lines of code have a direct representation on the screen. The following example draws a yellow rectangle on the screen:
size(200, 200); background(255); noStroke(); fill(255, 204, 0); rect(30, 20, 50, 50);
Continuous
This mode provides a setup() structure that is run once when the program
begins and a draw() structure which by default continually loops through
the code inside. This additional structure allows writing custom functions
and classes and using keyboard and mouse events.
This example draws four circles on the screen and utilizes a custom function
called circles(). The circles() function is not a part of the Processing
language, but was written for this example. The code in draw() only runs
once because noLoop() is called in setup().
void setup() { size(200, 200); noStroke(); background(255); fill(0, 102, 153, 204); smooth(); noLoop(); } void draw() { circles(40, 80); circles(90, 70); } void circles(int x, int y) { ellipse(x, y, 50, 50); ellipse(x+20, y+20, 60, 60); }
This example draws rectangles that follow the mouse position (stored in the system variables mouseX and mouseY). The draw() block runs forever until the program is stopped, thus creating the potential for motion and interaction.
void setup() { size(200, 200); rectMode(CENTER); noStroke(); fill(0, 102, 153, 204); } void draw() { background(255); rect(width-mouseX, height-mouseY, 50, 50); rect(mouseX, mouseY, 50, 50); }
Java
This mode is the most flexible, allowing complete Java programs to be written from inside the Processing Environment (as long as they're still subclasses of PApplet). This mode is for advanced users only and is not really recommended. Using this mode means that any additional tabs will no longer be inner classes, meaning that you'll have to do extra work to make them communicate properly with the host PApplet. It is not necessary to use this mode just to get features of the Java language.
public class MyDemo extends PApplet { void setup() { size(200, 200); rectMode(CENTER); noStroke(); fill(0, 102, 153, 204); } void draw() { background(255); rect(width-mouseX, height-mouseY, 50, 50); rect(mouseX, mouseY, 50, 50); } }
Rendering Modes
Processing currently has four rendering modes. The programs written with Processing can be rendered using the Java 2D drawing libraries, a custom 3D engine called P3D, and through OpenGL using the JOGL interface, and a custom 2D engine called P2D. The rendering mode is specified through the size() function. A large effort has been made to make the Processing language behave similarly across the different rendering modes, but there are currently some inconsistencies.
For more information, see the size() reference entry.
Applet Export
This topic is now discussed on the Processing wiki.
Application Export
This topic is now discussed on the Processing wiki.
Applet Security Restrictions
This topic is now discussed on the Processing wiki.
"Present" Features
This topic is now discussed on the Processing wiki.