15110 Summer 2017
Lab 12
Goals
In previous labs you've seen how to draw simple shapes on the canvas
and combine them to make interesting graphics like fractals. In this
lab you will continue with graphics, exploring how arbitrary images
can be encoded as two-dimensional list of pixels and manipulated
using iteration.
When you are done you should be able to do the following:
- Understand how a two-dimensional list of pixels maps to the
image on the screen.
- Create a list representing a simple image.
- Be fluent with code handling two-dimensional lists.
- Perform simple geometric transformations on an image
representation, like flipping horizontally or vertically.
- Perform simple color transformations on an image representation.
Files
Deliverables
- flip_image.py
- c_flag.py
- swap_bg.py
- grayscale.py
- transpose.py
Part 1 - Bitmap Images
1.1 Class Activity
- Create a lab12 directory. Download the files image.py and nautical.ppm
to this directory and cd to this directory.
Run python3 and display the nautical flag alphabet
on a Canvas by evaluating the following Python expressions:
from image import *
b = read_ppm("nautical.ppm")
c = start_image(250)
draw_image(c, b, 0, 0)
| |
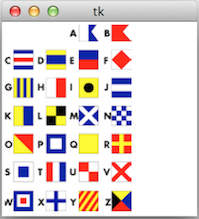 |
- The nautical alphabet image is a two-dimensional list of 200 by 136 pixels. It is encoded
as a list of lists. The main list contains 200 rows. Each row is
a list containing 136 pixels. Each pixel is a list of form
[r,g,b], where r, g, and b are red, blue, and green intensity values
from 0 to 255. Try the following expressions:
len(b)
len(b[0])
b[0][0]
b[100][100]
Knowing that an image is a list of rows, write a function
flip_image(image) that creates a new image that
is image flipped upside-down and plots the result. Put this function
in flip_image.py Then try flipping the nautical alphabet
image upside-down.
1.2 Student Activities
- In the file c_flag.py, write a function c_flag to create a little 5-by-6 pixel
image encoding the flag for the letter C in the nautical flag
alphabet. Refer to
the RGB
Color Table to get the codes for red and navy blue. Since this
image is very small, you will want to magnify it for display purposes
by writing draw_image(c, c_flag(), 0, 0, 20).
You may choose to do either 2 or 3. Your choice.
- In the file swap_bg.py, write a function swap_bg(image) that swaps the blue and
green values of every pixel in the image, and plots the result. Test
it on the nautical flag image. What happens if you call
swap_bg repeatedly on the same image?
- In the file grayscale.py, write a function grayscale(image) that converts an
image to grayscale by setting each pixel's r, g, and b values to their
average value (r+g+b) // 3, and plots the resulting grayscale image.
Test your function on the nautical flag image.
- Challenge problem: write a function transpose
that flips an image along the major diagonal, so that the rows become
columns and the columns become rows, and plots the result.
Below is an image of the expected output: