Homework 3 - Protocols, Delegates, and TinyReminders
In the past lecture, we learned about Protocols, Delegates, and special view controllers called NavigationViewControllers and TableViewControllers. Additionally, in class, we built a very minimal model of a Reminders app that uses both of those ViewControllers. In this homework, we'd like to build on all of those skills.
It's highly suggested that you start this homework early and ask for help ASAP. It isn't meant to be a hard homework, but it is a bit longer than the past two we've had. This homework is due on Wednesday, February 4th at 11:59 PM. Make sure to use office hours and email us if you have questions.
Receiving Homework Files
In order to receive your homework files, simply run (in your repo):
git pull
You should receive a hw3/ folder with the following contents: 1) HW3_Protocols_and_Delegates.playground/, a worksheet; and 2) TinyReminders/, a partially realized version of TinyReminders.
Make sure your repo has these files when you pull.
Hand-In
Remember, you can hand-in as many times as you like. In fact, incremental hand-ins are highly suggested so that you don't accidentally lose any work. But, just like in the last homework, you can submit your work with the submit.sh script. When you're in the root of your repo, simply type
./submit.sh
And everything should submit with no issues (of course, read the output to see if anything goes wrong--most times, you might just need to 'git pull' before you 'git push').
Part One: Protocols and Delegates Worksheet
When you pull your repo, you should see HW3_Protocols_and_Delegates.playground/. When you open this up with Xcode, you'll see a Playground with some questions and code samples. Just fill in the answers, and implement the code--pretty straightforward.
Part Two: Extending TinyReminders
When we built TinyReminders, we pre-programmed some reminders and watched as our app displayed our reminders in a Table View and in a different display view. For a refresher of the TinyReminders app, check it out here.
However, TinyReminders lacked very important functionality: the ability to add Reminders! Your job for HW 3 is to add this functionality. The app should run like this:
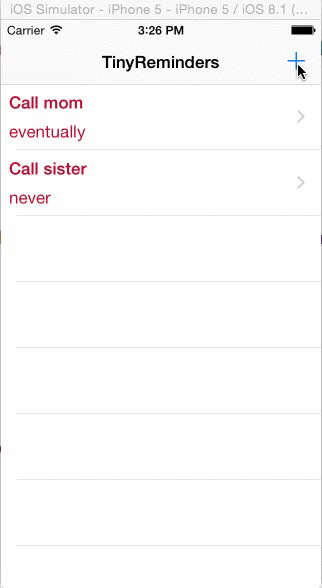
The above is an ideal example of how your HW submissions will work--so, your grade will be somehwat proportional to how much you can make your app function like the above. We won't walk you through the process this time though (like we did with PhraseThrow). Instead, we'll give you a reasonably descriptive list of steps to take and leave it to you to Google things or use the course staff because we're bored.
There's also opportunities for extra credit if you're super motivated. For now, though, just git pull and open up the TinyReminders project.
Step-by-Step
This step-by-step guide will tell you how to extend the partially completed TinyReminders in your repo to one that can add new reminders. If at any point a step is confusing to you (e.g. "What is a navigation stack?") come find us asap, either at OH or on FB.
1. Add a new ViewController to your Storyboard, and a new ViewController class to your project. This will represent the view where you will make a new reminder. Don't forget to link the new view to the new view controller class.
2. Add a Bar Button Item to your main table view and add a push segue (control-click-and-drag from the Bar Button Item to the new view controller; select push; give an identifier to this segue). Run your app and make sure this works. Do not proceed until this works. You should be able to print a "Hello, world!" in the new view controller's viewDidLoad whenever you move to the new view controller.
3. Add two text fields to your view. Link them to your view controller code.
4. Add a Bar Button Item to your new-reminder view controller and link it to your view controller code (as an action). There is no segue to pop a view controller off of the navigation stack--that's what the back button is for. The save button should pop the new-reminder view controller off of the stack and (eventually) pass information back to the main table view controller. You'll have to do this manually, and it's easy to do. In your function that is called when you press the save button, you can simply say:
@IBAction func saveNewReminder(sender: UIBarButtonItem) { // logic to send new reminder information down the stack. self.navigationController!.popViewControllerAnimated(true) }
That function above, popViewControllerAnimated is equivalent to hitting the Back button. Make sure that functionality works before continuing.
5. At this point, we need to think carefully about how we're going to pass information from our new-reminder view controller to our main table view controller. The nicest, clenaest way to do this is with Protocols and Delegates. In essence, your new-reminder view controller needs a helper to help it pass some information that it gathered to your main table view controller. This mirrors the Morbulator example on the website, where the new-reminder view controller is kind-of like your MorbulatorManager class. Make sure this makes sense to you before continuing.
6. Execute the delegation pattern to pass information back to your main table view controller and add a new Reminder to self.reminders. You can just define a new protocol in the same file as your new-reminder view controller. It doesn't matter how you do it (whether you pass a Reminder back, or two Strings back or whatever), just find a way to do it. Remember, before you segue to your new-reminder view controller, you can access that destination view controller in the prepareToSegue function--you can use this to set the delegate of your new-reminder view controller.
7. Your app should work now! If it doesn't, take a careful look through the error messages first. After you try to solve the problem by looking at the error messages come to Office Hours or message one of us on Facebook. Good luck!
Extra Credit
- Add the ability to delete reminders (look up deleting things from a table view controller).
- Add autolayout to the entire project.
- Add some color to the project--make it look nice!